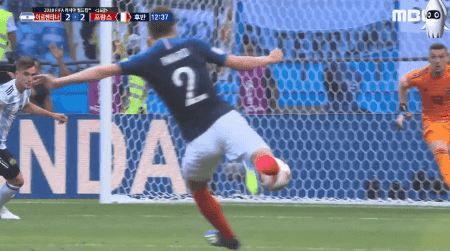
안드로이드 리사이클러뷰 아답터
Android RecyclerView Adapter
그런데 이거 마치 일기같다
It's a really popular recycle view instead of ListView, which was harder than ListView Adapter.
My teacher, I made Adapters through Google again, so I am still very immature.
When you get used to it, it's definitely a comfortable and good function, but it's still awkward.
But it's like a diary.
public class Menu_Adapter extends RecyclerView.Adapter<Menu_Adapter.MyViewHolder> {
private ArrayList<Category> mList;
private Context context;
public class MyViewHolder extends RecyclerView.ViewHolder {
protected TextView menu_name;
protected ImageView menu_image;
public MyViewHolder(View view) {
super(view);
this.menu_name = (TextView) view.findViewById(R.id.menu_name);
this.menu_image = (ImageView) view.findViewById(R.id.menu_image);
}
}
public Menu_Adapter(ArrayList<Category> list) {
this.mList = list;
}
// Create new views (invoked by the layout manager)
@Override
public Menu_Adapter.MyViewHolder onCreateViewHolder(ViewGroup parent,
int viewType) {
// create a new view
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.menu_item, parent, false);
MyViewHolder viewHolder = new MyViewHolder(view);
context = parent.getContext();
return viewHolder;
}
// Replace the contents of a view (invoked by the layout manager)
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
// - get element from your dataset at this position
// - replace the contents of the view with that element
holder.menu_name.setText(mList.get(position).getName());
Picasso.with(context)
.load(mList.get(position).getImage())
.into(holder.menu_image);
}
// Return the size of your dataset (invoked by the layout manager)
@Override
public int getItemCount() {
return (null != mList ? mList.size() : 0);
}
}
사실 아직도 메소드의 정확한 기능은 모르겠으나 어느정도 뭐 하는 역할인지는 알 것 같다.
위 Adapter는 기본으로 만들어지는 Adapter인데 내거에 맞게 다시 구글을 통하여 조금 고쳤다.
MyViewHolder에서는 findViewById를 하는 메소드인 것 같다. 여기서 모든 아이디를 통해 찾는다.
Menu_Adpater는 리스트를 받아 다시 이 Adpater의 리스트에 추가한다.
여기서 Category는 Getter/Setter이 있는 클래스이다.
onCreateViewHolder에서는 레이아웃을 메모리에 올리는 작업을 한다.
추가적으로 context를 필요로 할때 여기서 MyViewHolder viewHolder = new MyViewHolder(view);
context = parent.getContext() 을통해 context를 가져올 수 있다.
나는 Picasso를 사용 하기위해 가져왔는데 context가 필요로 하는 라이브러리를 사용할 때 위와 같은 방법으로 가져오면 context를 사용하기 하다. 이건 스택오버플로우에서 배웠다.
onBindViewHolder 메소드는 가져온 값을 set하는 곳인데, 바로 값을 set하지 않고 리스트에서 position을 통해 가져오도록 한다.
왜냐하면 리사이클러뷰에 한개만 보여줄거면 상관이 없는데 여러개의 자료를 보여줘야하면 반복을 해야하는데
이러한 반복을 해서 set해주는 메소드이기 때문이다.
getItemCount는 사이즈를 가져오는 메소드인 것 같은데..count를 가져와서..어디다가 써야할지 사실 아직 잘 모르겠다.
리사이클러뷰에 생성될 Item리스트에 상관이 있는 메소드일까 0이면 그만 만들어지도록 하기 위한?
In fact, I still don't know the exact function of the method, but I think I know what the role is.
The above adapter is a built-in adapter and I fixed it a little again through Google to suit my needs.
In MyViewHolder, it seems to be a method that does findViewById. Find it through all the IDs here.
Menu_Adpater receives the list and adds it back to this Adapter list.
where Category is a class with a Getter/Setter.
OnCreateViewHolder works by placing the layout in memory.
where MyViewHolder viewHolder = new MyViewHolder (view);
Context = content.getContext() can be obtained.
I brought it to use Picasso and when I use the library that I need it, I use Context if I bring it in the same way. I learned this from Stackoverflow.
The onBindViewHolder method is where you set the imported value, so that you do not set the value immediately and import it through the position in the list.
Because I don't care if you're going to show one to a re-cycleview, but if you're going to show me a bunch of data, I'm going to have to repeat it.
This is because it is a method that sets the method by repeating iteration.
I think getItemCount is a method that brings size.I've got a count.I don't really know what to use yet.
Is the method relevant to the list of items to be created in the Recycle Bin or to make sure zero is not created?
recycler_menu = (RecyclerView) findViewById(R.id.recycler_menu);
recycler_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycler_menu.setLayoutManager(layoutManager);
mArrayList = new ArrayList<Category>();
dto = new Category();
category.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
for (DataSnapshot bring : dataSnapshot.getChildren()) {
dto = new Category();
menu_SetName = bring.child("Name").getValue().toString();
menu_SetImage = bring.child("Image").getValue().toString();
dto.setName(menu_SetName);
dto.setImage(menu_SetImage);
Log.d("또 확인2222 ", dto.getName());
mArrayList.add(dto);
}
adapter = new Menu_Adapter(mArrayList);
recycler_menu.setAdapter(adapter);
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {}
});
recycler_menu = (RecyclerView) findViewById(R.id.recycler_menu);
recycler_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycler_menu.setLayoutManager(layoutManager);
을 통해 리사이클러뷰를 정의하는 부분이다. 저번에 모르고 Manager을 set하지 않아서 계속 오류가 났던 기억이 난다.
위에 코드는 파이어베이스에서 데이터를 받는 코드인데,
중요한건 dto에 set하고 그것을 여기서 만든 List에 add해서 Adpater에 넘기는 것이 중요하다.
그리고 Adapter을 리사이클러뷰에 set하면 끝이다.
참고로 반복문안에서 dto를 생성해줘야지 반복문 밖에서 생성해버리면 계속 마지막 데이터만 출력이 된다.
이거 알아내느라 조금 고생했다..쉬운거였는데
recycler_menu = (RecyclerView) findViewById(R.id.recycler_menu);
recycler_menu.setHasFixedSize(true);
layoutManager = new LinearLayoutManager(this);
recycler_menu.setLayoutManager(layoutManager);
The part that defines the Recycle Bin view through . I remember that I didn't set the manager without knowing it the other day, so I kept getting the error.
The code above is the code that receives data from the Firebase.
It is important that you set the dto and add it to the list that you made here and hand it over to the Adpater.
And if you set the Adapter in the Recycle Bin, it's over.
Note that dto should be generated from the iterative statement, if it is created outside the iterative statement, only the last data will still be output.
I had a hard time finding out about this.It was easy.