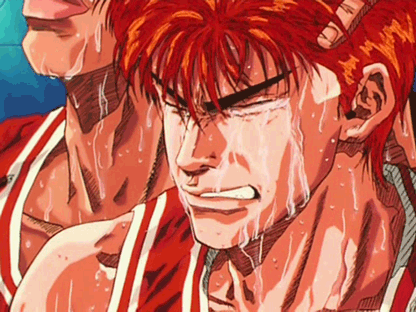
안드로이드 파이어베이스를 이용한 회원가입 및 로그인
Sign up and log in using Android Firebase
웹을 공부할때는 오라클DB을 이용하여 쿼리를 가지고 회원가입 및 로그인 처리를 하였는데.,
안드로이드로 넘어오면서 파이어베이스를 많이 사용한다고 하여 파이어베이스를 이용한 회원가입 및 로그인을 해봤다.
물론 안드로이드에서도 서버를 통하여 오라클DB등과 연결하여 처리할 수 있지만,
굳이 구글에서 더 간단하게 만들어 놓은 파이어베이스를 내비두고 그럴 필요가 있을까 싶다.
그리고 어디서 들은 강의에서도 정말 큰 회사가 아닌이상 많은 회사들이 파이어베이스를 이용한다고 한다.
When I was studying the web, I used OracleDB to sign up and log in with a query.
As I moved on to Android, I signed up and signed up using FireBase because used FireBase a lot.
Of course, Android can also connect and process OracleDB and so on through the server.
I wonder if there is a need to do so without mentioning the simpler version of the Firebase made by Google.
And many companies use FireBase as long as it's not really a big company in lectures I've taken somewhere.
일단 파이어베이스 데이터베이스에 Json형태로 간단하게 테이블을 만들었다.
아직 제대로 할 줄 몰라 objZen인가를 사용하여 만들었다.
Once in the Firebase database, we simply created a table in Json format.
I didn't know how to do it yet, so I made it using the objZen accreditation.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sign_up);
edtPhone = (MaterialEditText) findViewById(R.id.edtPhone);
edtName = (MaterialEditText) findViewById(R.id.edtName);
edtPassword = (MaterialEditText) findViewById(R.id.edtPassword);
btnSignUp = (Button) findViewById(R.id.btnSingUp);
// Init firebase
final FirebaseDatabase database = FirebaseDatabase.getInstance();
final DatabaseReference table_user = database.getReference("User");
btnSignUp.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final ProgressDialog mDialog = new ProgressDialog(SignUp.this);
mDialog.setMessage("Please Waiting");
mDialog.show();
table_user.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
// check if already user phone
if (dataSnapshot.child(edtPhone.getText().toString()).exists())
{
mDialog.dismiss();
Toast.makeText(SignUp.this, "already register", Toast.LENGTH_LONG).show();
}
else
{
mDialog.dismiss();
UserDTO user = new UserDTO(edtName.getText().toString(), edtPassword.getText().toString());
table_user.child(edtPhone.getText().toString()).setValue(user);
Toast.makeText(SignUp.this, "sign up successfully", Toast.LENGTH_LONG).show();
finish();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
});
}
먼저 파이어베이스에 접근하기 위해 FirebaseDatabase database = FirebaseDatabase.getInstance(); 과
DatabaseReference table_user = database.getReference("User");을 정의한다.
database.getReference("User")의 User은 Json의 형태로된 데이터베이스에서 가장 큰 부모객체를 정확히 쓴다.
PhoneNumber이 PrimaryKey이기 때문에 PhoneNumber로 구분을 한다.
exists()는 존재한다면..이라는 건데 true라면 회원가입이 이미 되었다고 한다. 반대로 false라면 다음으로 넘어간다.
미리 만들어둔 UserDTO에 입력받은 이름과 비밀번호를 넘기고 .setValue()를 통해 입력받은 핸드폰 번호와 DTO에 담긴
이름과 비밀번호를 함께 전달한다.
그리고 제대로 되었더라면 다시 파이어베이스 사이트로 가서 확인을 해본다면 제대로 입력이 되었을 것이다.
First, define FirebaseDatabase database = FirebaseDatabase.getInstance(); and DatabaseReference table_user = database.getReference("User"); to access the Firebase.
The user in database.getReference ("User") accurately writes the largest parent object in the database in the form of Jason.
Because PhoneNumber is the PrimaryKey, it is classified as PhoneNumber.
If exists() exist...It's called true, but it's already registered. On the other hand, if it's false, it goes on to the next one.
The name and password entered in the pre-created UserDTO are passed, and the mobile phone number entered through the .setValue() is included in the DTO Deliver the name and password together.
And if it had worked, if you could go back to the Firebase site and check it out, it would have been done.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_signin);
edtPhone = (MaterialEditText) findViewById(R.id.edtPhone);
edtPassword = (MaterialEditText) findViewById(R.id.edtPassword);
btnSignIn = (Button) findViewById(R.id.btnSingIn);
// Init Firebase
FirebaseDatabase database = FirebaseDatabase.getInstance();
final DatabaseReference table_user = database.getReference("User");
btnSignIn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final ProgressDialog mDialog = new ProgressDialog(Signin.this);
mDialog.setMessage("Please Waiting");
mDialog.show();
table_user.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot) {
// check if user not exist in database
if (dataSnapshot.child(edtPhone.getText().toString()).exists()) {
// Get user information
mDialog.dismiss();
UserDTO user = dataSnapshot.child(edtPhone.getText().toString()).getValue(UserDTO.class);
if (user.getPassword().equals(edtPassword.getText().toString())) {
//Toast.makeText(Signin.this, "Sign in seuccessfully", Toast.LENGTH_SHORT).show();
Intent homeIntent = new Intent(Signin.this,Home.class);
Common.currentUsers = user;
startActivity(homeIntent);
finish();
} else {
Toast.makeText(Signin.this, "Sign in failed!!!", Toast.LENGTH_SHORT).show();
}
}
else
{
mDialog.dismiss();
Toast.makeText(Signin.this, "User nt exist in Database", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onCancelled(@NonNull DatabaseError databaseError) {
}
});
}
});
로그인도 마찬가지로 파이어베이스와 데이터베이스에 접근부터 한다.
이번엔 DTO에 입력받은 전화번호와 그에 따른 자식들을 데리고 와서 넘긴다.
그리고 DTO를 이용하여 DTO에 넘긴 비밀번호와 입력받은 비밀번호가 같은지 확인을 한후 작업을 진행한다.
Logins also access the Firebase and database first.
This time, the phone number entered in the DTO and the corresponding children are brought and handed over.
Then, use DTO to verify that the password passed to DTO is the same and then proceed with the operation.
ps 1. 나도 이번에 처음해봐서 설명이 많이 빈약함
2. 그렇다고 다른 게시물의 설명이 충분한것도 아님..ㅠ